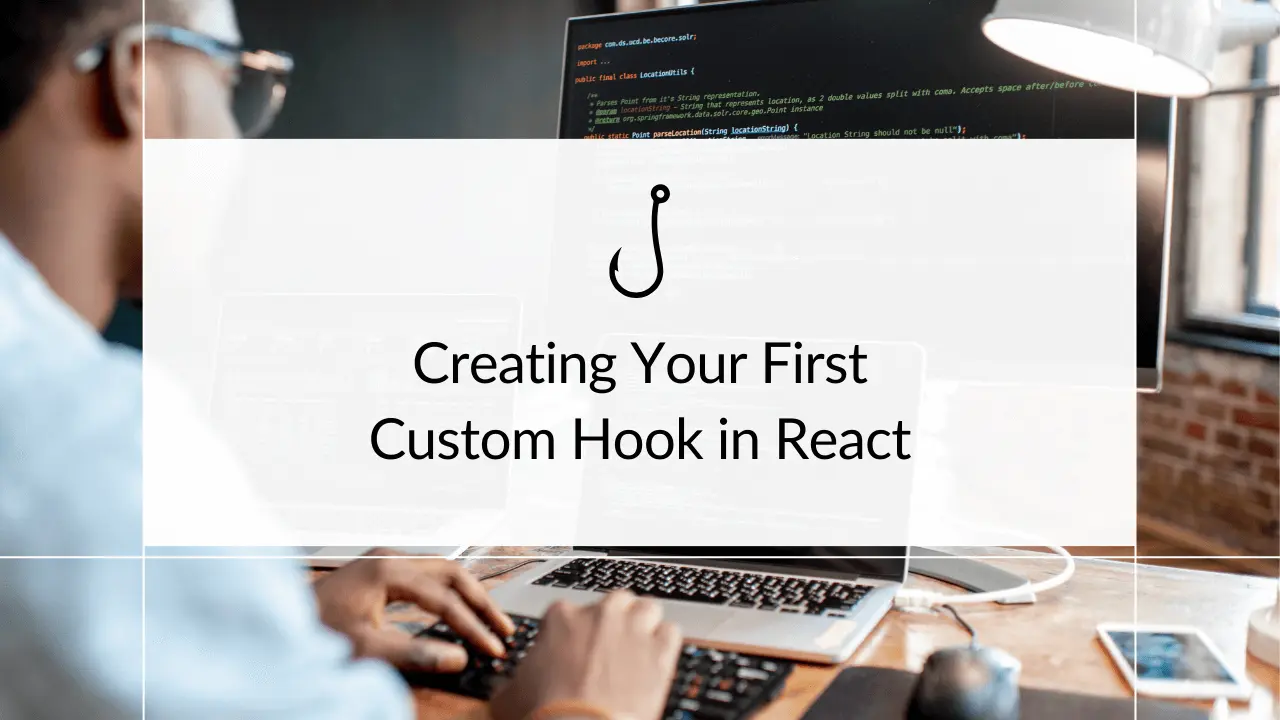
Creating Your First Custom Hook in React
react javascript front-end
Custom Hooks provides a more intuitive and functional approach to state management and side effects in React. This article is a step-by-step guide on creating custom Hooks that encapsulate logic and state, making your components more maintainable and reusable.
Why Custom Hooks?
Custom Hooks allow you to abstract component logic into reusable functions. This makes your codebase cleaner, helps avoid repeating code, and facilitates easier testing. Let’s dive into how to create a simple custom React Hook.
Creating Your First Custom Hook: useFormInput
Setup and Initial State
We’ll start by creating a Hook that manages the state of a form input. Here’s a basic setup:
import { useState } from "react";
function useFormInput(initialValue) {
const [value, setValue] = useState(initialValue);
const handleChange = (event) => {
setValue(event.target.value);
};
return {
value,
onChange: handleChange,
};
}
Using useFormInput
in a Component
With useFormInput
, you can now manage form inputs with ease. Here’s how to use it in a component:
function MyForm() {
const username = useFormInput("");
return (
<form>
<input type="text" {...username} />
</form>
);
}
Beyond Basics: Creating a useFetch
Hook
Let’s create a more complex Hook, useFetch, which handles API calls.
Implementing useFetch
import { useState, useEffect } from "react";
function useFetch(url) {
const [data, setData] = useState(null);
const [loading, setLoading] = useState(true);
const [error, setError] = useState(null);
useEffect(() => {
const fetchData = async () => {
try {
const response = await fetch(url);
const result = await response.json();
setData(result);
setLoading(false);
} catch (error) {
setError(error);
setLoading(false);
}
};
fetchData();
}, [url]);
return { data, loading, error };
}
Utilizing useFetch
in a Component
function UserData() {
const { data, loading, error } = useFetch("https://api.example.com/users");
if (loading) return <div>Loading...</div>;
if (error) return <div>Error: {error.message}</div>;
return (
<ul>
{data.map((user) => (
<li key={user.id}>{user.name}</li>
))}
</ul>
);
}
Conclusion: The Power of Custom Hooks
Custom Hooks are a powerful feature in React for abstracting and reusing functionality. They help keep your components clean and your code DRY. I encourage you to experiment with creating your own Hooks based on your projects’ needs.